Coding Note
등록,수정, 조회 API 만들기! 본문
등록, 수정, 조회 API 만들기!
API를 만들기 위해 총 3개의 클래스가 필요하다!
1. Request 데이터를 받을 DTO
2. API 요청을 받을 Controller
3. 트랜잭션, 도메인 기능 간의 순서를 보장하는 Service
1. Spring 웹 계층
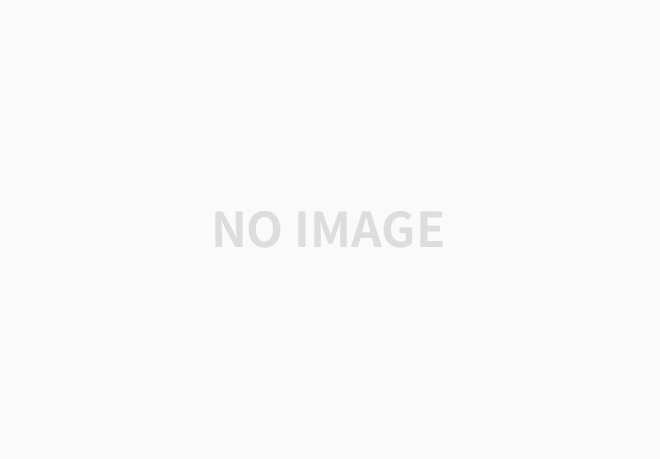
Web Layer
- 컨트롤러와 JSP/Freemaker 등의 뷰 템플릿 영역
- 필터, 인터셉터, 컨트롤러 어드바이스등 외부 요청과 응답에 대한 전반적인 영역을 이야기함.
Service Layer
- @Service에 사용되는 서비스 영역
- 일반적으로 Controller와 Dao의 중간 영역에서 사용됨
- @Transactional이 사용되어야 하는 영역
Repository Layer
- 데이터 저장소에 접근하는 영역
DTOs
- DTO(Data Transfer Object)는 계층 간에 데이터 교환을 위한 객체를 이야기하며 DTOs는 이들의 영역을 얘기함.
Domain Model
- 도메인이라 불리는 개발 대상을 모든 사람이 동일한 관점에서 이해할 수 있고 공유할 수 있도록 단순화시킨 것
- 비지니스 처리를 담당
2. 등록/수정/조회 API 만들기
아래 사진과 동일하게 디렉토리 및 클래스를 생성한다.
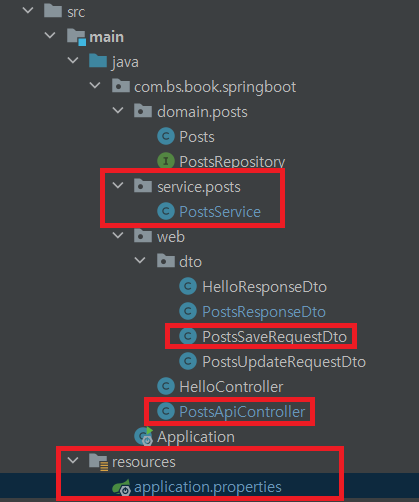
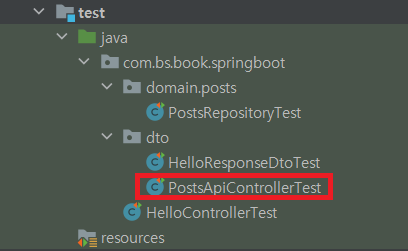
그럼 코드를 작성해보자!
2-1. 등록
- PostApiController
package com.bs.book.springboot.web;
import com.bs.book.springboot.service.posts.PostsService;
import com.bs.book.springboot.web.dto.PostsResponseDto;
import com.bs.book.springboot.web.dto.PostsSaveRequestDto;
import com.bs.book.springboot.web.dto.PostsUpdateRequestDto;
import lombok.RequiredArgsConstructor;
import org.springframework.web.bind.annotation.*;
@RequiredArgsConstructor
@RestController
public class PostsApiController {
private final PostsService postsService;
//등록
@PostMapping("/api/v1/posts")
public Long save(@RequestBody PostsSaveRequestDto requestDto) {
return postsService.save(requestDto);
}
}
- PostService
package com.bs.book.springboot.service.posts;
import com.bs.book.springboot.domain.posts.Posts;
import com.bs.book.springboot.domain.posts.PostsRepository;
import com.bs.book.springboot.web.dto.PostsResponseDto;
import com.bs.book.springboot.web.dto.PostsSaveRequestDto;
import com.bs.book.springboot.web.dto.PostsUpdateRequestDto;
import lombok.RequiredArgsConstructor;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@RequiredArgsConstructor
@Service
public class PostsService {
private final PostsRepository postsRepository;
//등록
@Transactional
public Long save(PostsSaveRequestDto requestDto) {
return postsRepository.save(requestDto.toEntity()).getId();
}
}
< 스프링에서 Bean을 주입 받는 방식 >
- @Autowired
- setter
- 생성자
가장 권장하는 방식은 생성자로 주입하는 것!
위 코드 또한 생성자를 통해 주입을 받은 것이다!
@RequiredArgsConstructor
- 롬복 어노테이션
- 새로운 서비스, 기존 컴포넌트를 제거하는 등의 상황이 발생해도 생성자 코드를 수정할 필요가 없음.
- PostsSaveRequestDto
package com.bs.book.springboot.web.dto;
import com.bs.book.springboot.domain.posts.Posts;
import lombok.Builder;
import lombok.Getter;
import lombok.NoArgsConstructor;
@Getter
@NoArgsConstructor
public class PostsSaveRequestDto {
//사용할 컬럼
private String title;
private String content;
private String author;
@Builder
public PostsSaveRequestDto(String title, String content, String author) {
this.title = title;
this.content = content;
this.author = author;
}
public Posts toEntity() {
return Posts.builder()
.title(title)
.content(content)
.author(author)
.build();
}
}
Entity클래스
- Request/Response 클래스로 사용 불가!
- 데이터베이스와 맞닿은 핵심 클래스!
- Entity 클래스 기준으로 테이블이 생성되고, 스키마가 변경됨
- Posts
package com.bs.book.springboot.domain.posts;
import lombok.Builder;
import lombok.Getter;
import lombok.NoArgsConstructor;
import javax.persistence.*;
@Getter
@NoArgsConstructor
@Entity
public class Posts {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(length =500, nullable = false)
private String title;
@Column(columnDefinition = "TEXT", nullable = false)
private String content;
private String author;
@Builder
public Posts(String title, String content, String author) {
this.title = title;
this.content = content;
this.author = author;
}
}
- PostRepository
package com.bs.book.springboot.domain.posts;
import org.springframework.data.jpa.repository.JpaRepository;
public interface PostsRepository extends JpaRepository<Posts, Long> {
}
- PostApiControllerTest
@RunWith(SpringRunner.class)
//JPA 기능까지 한번에 테스트할 때 사용
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class PostsApiControllerTest {
@LocalServerPort
private int port;
//JPA 기능까지 한번에 테스트할 때 사용
@Autowired
private TestRestTemplate restTemplate;
@Autowired
private PostsRepository postsRepository;
@After
public void tearDown() throws Exception {
postsRepository.deleteAll();
}
@Test
public void Posts_등록된다() throws Exception {
//given
String title = "title";
String content = "content";
PostsSaveRequestDto requestDto = PostsSaveRequestDto.builder()
.title(title)
.content(content)
.author("author")
.build();
String url = "http://localhost:" + port + "/api/v1/posts";
//when
ResponseEntity<Long> responseEntity = restTemplate.postForEntity(url,requestDto,Long.class);
//then
assertThat(responseEntity.getStatusCode()).isEqualTo(HttpStatus.OK);
assertThat(responseEntity.getBody()).isGreaterThan(0L);
List<Posts> all = postsRepository.findAll();
assertThat(all.get(0).getTitle()).isEqualTo(title);
assertThat(all.get(0).getContent()).isEqualTo(content);
}
}
< Posts 등록 API테스트 결과 >
- @SpringBootTest(webEnvironment = SpringBootTest.)
- WebEnvironment.RANDOM_PORT로 랜덤 포트 실행과 insert 쿼리가 실행된 걸 모두 확인!
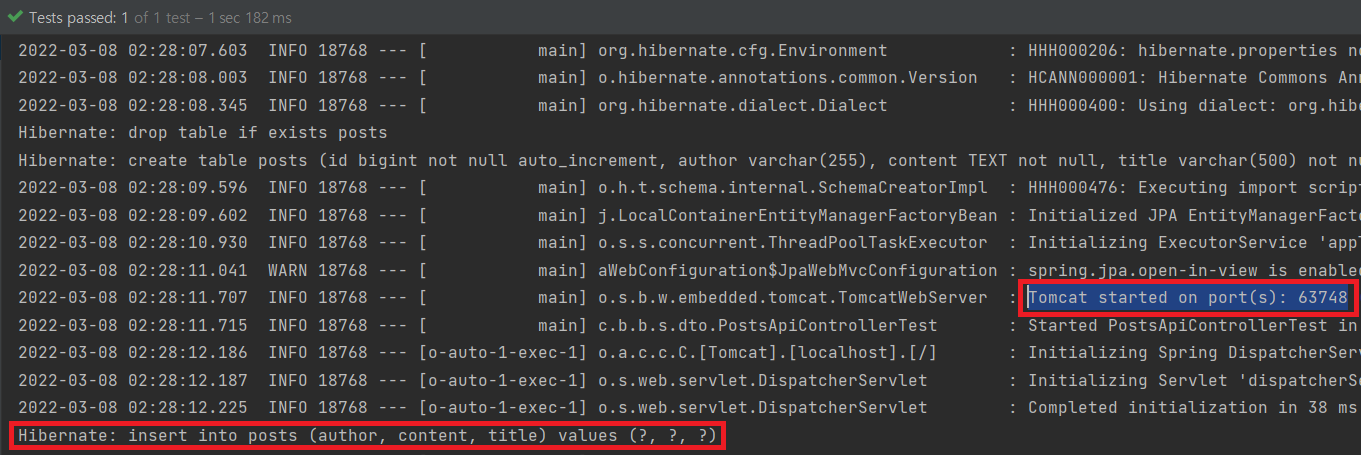
2-2. 수정
아래와 동일하게 각 파일에 수정 코드 추가하기
- PostApiController
//수정
@PutMapping("/api/v1/posts/{id}")
public Long Update(@PathVariable Long id, @RequestBody PostsUpdateRequestDto requestDto) {
return postsService.update(id, requestDto);
}
- PostsUpdateRequestDto
package com.bs.book.springboot.web.dto;
import lombok.Builder;
import lombok.Getter;
import lombok.NoArgsConstructor;
@Getter
@NoArgsConstructor
public class PostsUpdateRequestDto {
private String title;
private String content;
@Builder
public PostsUpdateRequestDto(String title, String content){
this.title = title;
this.content = content;
}
}
-Posts
public void update(String title, String content) {
this.title = title;
this.content = content;
}
- PostService
//수정
@Transactional
public Long update(Long id, PostsUpdateRequestDto requestDto) {
Posts posts = postsRepository.findById(id)
.orElseThrow(() -> new IllegalArgumentException("해당 게시글이 없습니다. id ="+id));
posts.update(requestDto.getTitle(), requestDto.getContent());
return id;
}
< Posts 등록 API테스트 결과 >
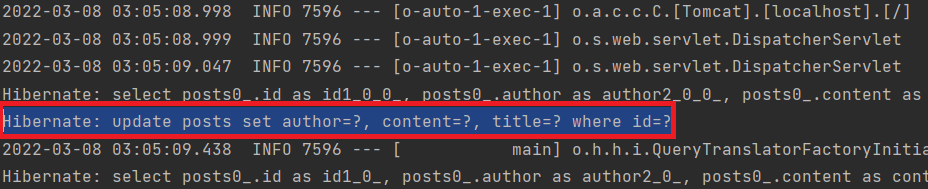
2-3. 조회
- PostApiController
//조회
@GetMapping("/api/v1/posts/{id}")
public PostsResponseDto findById (@PathVariable Long id) {
return postsService.findById(id);
}
- PostService
//조회
public PostsResponseDto findById (Long id) {
Posts entity = postsRepository.findById(id)
.orElseThrow(() -> new IllegalArgumentException("해당 게시글이 없습니다. id="+id));
return new PostsResponseDto(entity);
}
- PostApiControllerTest
@Test
public void Posts_수정된다() throws Exception {
//given
Posts savedPosts = postsRepository.save(Posts.builder()
.title("title")
.content("content")
.author("author")
.build());
Long updateId = savedPosts.getId();
String expectedTitle = "title2";
String expectedContent = "content2";
PostsUpdateRequestDto requestDto = PostsUpdateRequestDto.builder()
.title(expectedTitle)
.content(expectedContent)
.build();
String url ="http://localhost:" + port + "/api/v1/posts/" +updateId;
HttpEntity<PostsUpdateRequestDto> requestEntity = new HttpEntity<>(requestDto);
//when
ResponseEntity<Long> responseEntity =restTemplate.exchange(url, HttpMethod.PUT,
requestEntity, Long.class);
//than
assertThat(responseEntity.getStatusCode()).isEqualTo(HttpStatus.OK);
assertThat(responseEntity.getBody()).isGreaterThan(0L);
List<Posts> all = postsRepository.findAll();
assertThat(all.get(0).getTitle()).isEqualTo(expectedTitle);
assertThat(all.get(0).getContent()).isEqualTo(expectedContent);
}
2-4. 톰캣에서 실행해보기
데이터베이스로 h2 사용
- application.poperties에 다음과 같이 추가한다!
spring.h2.console.enabled=true
정상적으로 실행된걸 확인하기 위해 application의 main 메소드를 실행한다.
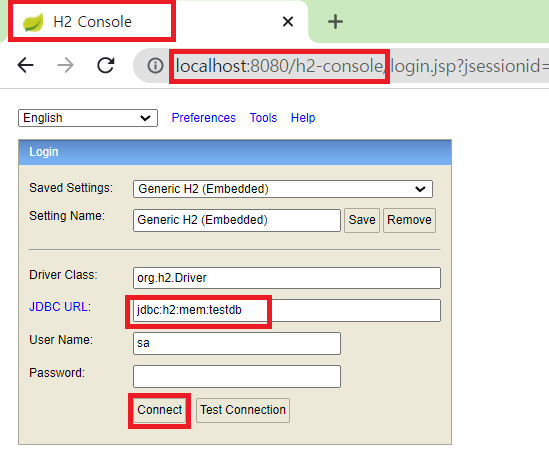
접속되면 URL를 사진과 동일하게 작성하고
Connect 버튼을 누르면 POSTS 테이블이 정상적으로 노출되는걸 확인할 수 있다!!
- 조회 쿼리 실행
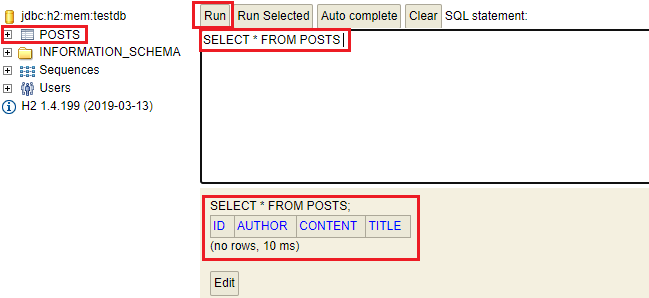
- 등록 쿼리 실행
insert 구문을 작성하고 실행하면 오류없이 출력되는 걸 확인할 수 있다.

등록된 데이터를 API를 요청해보자!
브라우저에 아래와 같이 입력해 조회 기능을 테스트 한다.
- 조회
http://localhost:8080/api/v1/posts/1
첫 결과는 오류였다... 왜!!!!!!!!!!
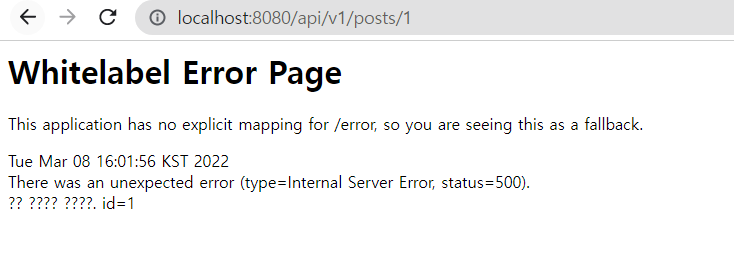
콘솔에서 에러 확인 후 코드 점검하고.. 오타 수정했는데도 오류ㅠㅠ
쉬고 다시 실행해보니 잘 작동했다..!
결론!
오타 수정하고 프로그램 껐다 켜보자!
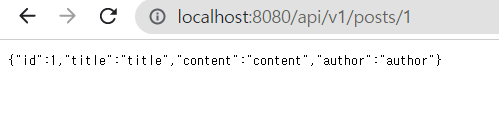
완성!!
'SpringBoot > AWS_PJ' 카테고리의 다른 글
머스테치로 화면 구성하기! (0) | 2022.03.09 |
---|---|
JPA Auditing으로 생성 시간/수정 시간 자동화하기! (0) | 2022.03.08 |
JPA로 데이터베이스 다루기! (0) | 2022.03.08 |
JPA 소개하기 및 데이터 베이스 다루기 (0) | 2022.03.07 |
Lombok 설치하기 (0) | 2022.03.01 |